Kaboum v4
Javascript Tools Application Programing Interface
Topic |
Kaboum Javascript tools API. (tools to deal with Kaboum in HTML pages) |
Author |
Nicolas Ribot |
Creation date |
July, 3rd 2005 |
History |
- v4_0_7 : Integration of Javascript code in the Kaboum (v4) project. History for previous changes in these JS files not kept
|
Table of Content
1. Description
2. Quick Guide for Javascript Integration
3. Javascript Files
4. Available Map Controls
5. Kaboum.js Reference

1. Description 
Kaboum is an applet written in java 1.1. It's working with web browser that conform to java
and javascript 1.1 specifications (including LiveConnect).
It is important to understand how Kaboum works, by first reading its API.
Kaboum receives and sends commands by a LiveConnect mechanism to Javascript code. To ease the use of the applet and its integration by developers/web admins into Web pages, a set of Javascript methods and objects were developped.
For example, the toolbar shown in the Figure 1. can be easily built with the Javascript API, using corresonping objects and methods.
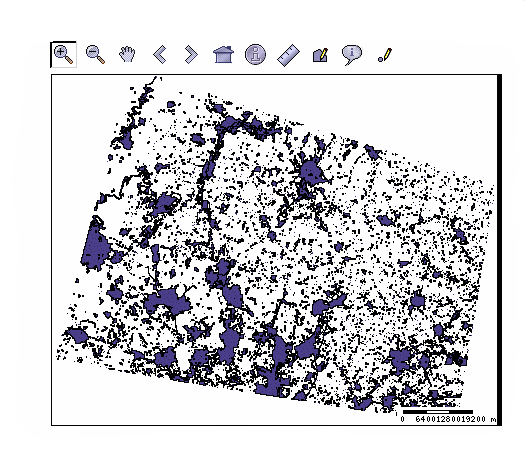
Figure 1 : global view. The toolbar is an HTML component
used to switch operating
modes. Command are send to the applet using javascript Kaboum API .
1.1. Fondamental Kaboum methods
Switching between operating mode is done using the Kaboum public method kaboumCommand(String command). This method can be invoqued by Javascript by calling it on the kaboum applet object, once defined in the HTML page.
On return, applet sends message to the web browser by calling the javascript method
kaboumResult(String result). This method must exist in the document containing the applet in order for Web page to capture and handle Kaboum messages.
The Javascript API handles these methods to send and receive Kaboum commands.
N.B. : this communication process works only if LiveConnect is enabled for the target browser.

2. Quick guide for Javascript integration
The best way to integrate Kaboum Javascript API with the Kaboum applet is to read all this document to understand available objects and methods.
For those in a hurry, here is a quick guide to help you start with Javascript integration:
First, design the web page that will contain the Kaboum applet. See the Kaboum API for applet initialisation parameters and other parameters. Creates the applet tag in your page where you want to see the map. The applet MUST BE NAMED "kaboum".
Second, link the Kaboum Javascript file: <SCRIPT language=JavaScript src="<script folder>/kaboum.js"></SCRIPT>
Third, defines a <form> element for the page. You can define it globally, just after the <body> element, and close it just before </body>
Fourth, find the places in your HTML code where you want to integrate the toolbar, the layer control, the scale control, the legend control, etc.
Fifth, includes the Javascript methods in these places, be sure to understant their parameters
For the toolbar:
<script>
kNewButton('images/zoomin', 'Zoom In', 'modal', 'ZOOMIN', 22, 22);
kNewButton('images/zoomout', 'Zoom out', 'modal', 'ZOOMOUT', 22, 22);
kNewButton('images/pan', 'Pan the map', 'modal', 'PAN', 22, 22);
kNewButton('images/back', 'previous map location', 'action', 'BACK', 22, 22);
kNewButton('images/forward', 'next map location', 'action', 'FORWARD', 22, 22);
kNewButton('images/home', 'Return to home page', 'action', 'HOME', 22, 22);
kNewButton('images/info', 'Layers information', 'modal', 'QUERY', 22, 22);
kNewButton('images/distance', 'measure distances', 'modal', 'DISTANCE', 22, 22);
</script>
For the layer controls :
<script>
kNewTheme("departements","Départements Français",true,true,"CHECKBOX",'tiny');
kNewTheme("roads","Routes",true,true,"CHECKBOX",'tiny');
kNewTheme("rivers","Rivieres",true,true,"CHECKBOX",'tiny');
</script>
For the scale:
<script>kNewScale("1:","");</script>
For the legend (a pop windows will be opened, containing the Mapserver legend, provided a LEGEND object was defined in the mapfile):
<a href="#" onclick="kGetLegend();">Display the map legend</a>
You're done ! Refer to the test page for further examples.

3. Javascript files
Two Javascript files contain all the variables, objects and methods needed to deal with the Kaboum Applet:
- kaboum.js: contains all the code to deal with Mapserver Kaboum features (layer control, toolbars, legends, scale, etc.)
- kaboum_edition.js: contains all the code to deal with advanced edition features provided by kaboum (loading vector objects, dealing with servers, etc.). This file should be included into the web page containing kaboum only if vector objects edition features are used in kaboum. Otherwise, it is useless.
As a general guideline, these files must not be edited or changed. Instead, developpers are encouraged to add their own Javascript code (as a file or directly into the Web page) and to overload variables/methods if needed in order to achieve particular tasks.
3.1. Integrating Javascript files in the Kaboum web page
The easiest way to integrate Javascript files into the page containing kaboum is to link these files into a <script> tag, as shown below:
<SCRIPT language=JavaScript src="<script folder>/kaboum.js"></SCRIPT>
<SCRIPT language=JavaScript src="<script folder>/kaboum_edition.js"></SCRIPT>
where <script folder> is the folder (relative or absolute URL) containg the 2 files
Note: Kaboum Javascript needs a <form> object to be included in the page in order for the methods to work. The form can have any name.

4. Available Map Controls
Kaboum's purpose is (roughly) to display a single Mapserver map in its windows. The applet itself contains nothing but the map. To interact with the map, change kaboum modes, control map layers, display some information, etc. it is necessary to include some Javascript code doing the tricks.
The Kaboum Javascript files provides methods and variables to achieve this. Especially, the following logical components are available through Javascript code:
- The kaboum commands control (often called toolbar)
- The layers control
- The legend control
- The scale control
- The coordinates control
4.1 The kaboum commands control
4.1.1 Introduction
Kaboum's commands (zoom, pan, home, back, forward, print, etc...) can be represented by buttons that can be grouped together into toolbar(s). Kaboum Javascript API ease the creation of such buttons by providing methods to create buttons.
Buttons can be dynamic, ie having a different face according to their state (clicked, hovered, normal). To do that, a set of 3 buttons must be defined for each command. Their name is important. For instance, for a zoomin command if the main image is zoomin.gif, then zoomin_clicked.gif and zoomin_over.gif must be created, representing the state of the button when it is clicked and hovered. The "_clicked" and "_over" keywords are automatically added by the API when dealing with buttons.
Of course, one can defined 3 identical buttons if static behaviour is needed.
Here is an example of the provided buttons for the zoomin mode: zoomin.gif, zoomin_clicked.gif, zoomin_over.gif

Kaboum commands can be divided into 2 groups: modal and action commands:
- Modal command sets kaboum to a certain mode until a new mode is sent to kaboum (example: zoom mode)
- Action command executes an action immediately. Kaboum stays in the mode it was before the action is executed (example: back action)
Commands control API allows to create both types of buttons: modal or action.
4.2.2 How to create a command button: the kNewButton(...) method
To add a new button in the kaboum page corresponding to a command, use the following Javascript method:
kNewButton(img_src, alt_txt, mode, action, img_width, img_height):
Description |
Creates a new button by generating HTML code according to given parameters. The generated button will send command to kaboum each time it is clicked. According to the button's mode, kaboum will change its mode or execute the button's action |
Parameters |
img_src:
Type |
String. |
Description |
The URL to the image for this button. Do not add file extension. It is automatically generated by the API according to the kButtonType global parameter. |
Example |
'images/zoomin' |
alt_txt:
Type |
String. |
Description |
The text to display when mouse is over the button. |
Example |
'Zoom in mode' |
mode:
Type |
String. |
Description |
The type of the button, either modal or action |
Example |
'modal' or 'action' |
action:
Type |
String. |
Description |
The kaboum command to send when clicking this button. Refer to the kaboum API for the list of supported commands |
Example |
'ZOOMIN' or 'PAN' or... |
img_width:
Type |
Integer |
Description |
The width of the images for this button, in pixels. (provided images are 22 px). |
Example |
22 |
img_height:
Type |
Integer |
Description |
The height of the images for this button, in pixels. (provided images are 22 px). |
Example |
22 |
|
example |
<script>kNewButton('images/zoomin', 'Zoom In mode', 'modal', 'ZOOMIN', 22, 22);</script>
|
|
4.2 The layers control
4.2.1 Introduction
Mapserver organizes the geographic data into layers. Each layer contains a consistent set of geographic objects (points, lines, polygones, rasters). Each layer has a set of properties (the way it is drawn, its behaviour, its projection, etc.).
In a map environment, it can be powerfull to allow user to compose his own map by selecting/deselecting some layer, turning information feature on or off for certain layers, change the map background from a satellite image to a topographic scan, and so on.
Kaboum Javascript API eases the creation of layer controls inside the web page by providing a method (generating the Javascript code behind the scene) to add a layer control anywhere in the Web page
4.2.2 How to create a layer control for kaboum: the kNewTheme(...) method
To add a layer control in the web page containing kaboum, you must first decide the type of Javascript component that will represent the the layer. Currently, you can choose either a select component or a checkbox component.
- Select component is an handy way to provide user with a way to swap map background, when only one background is pertinent at a time. (swapping from satellite image to scan map or orthophotgraphy).
When defining a select element (<option> tag), the developer must use a function when the last select element is defined, in order for the code to close the <select> tag correctly. This method is named: kLastSelectTheme()
- Checkbox(es) component(s) is(are) nice to handle several vector layers as they appear in the MapServer Mapfile. With them, you can toggle layer visibility and toggle kaboum layer information command on a per-layer basis.
Then use the method below to define the layer control:
kNewTheme(name, display_name, visible, info, type, css_style):
Description |
Creates a layer control, (either a checkbox or a select entry, depending on the type parameter) by generating HTML code according to given parameters. The generated control will allow user to toggle layer visibility when selecting or checking it. This default behaviour (automatic layer refresh) can be controled by the kThemeAutoReload global variable. True by default). |
Parameters |
name:
Type |
String |
Description |
The name of the Mapserver layer (or group name) as it appears in the mapfile. This name is sent by kaboum to mapserver to set the list of visible layers. |
Example |
'departements' |
display_name:
Type |
String. |
Description |
The name of the layer (or any other text) as it will be displayed in the html page, either next to the checkbox or in the select component, according to the "type" parameter |
Example |
'Départements Français' |
visible:
Type |
boolean |
Description |
True to make the layer visible by default when kaboum loads the first map. False to hide the layer |
Example |
|
info:
Type |
boolean |
Description |
Indicates if a layer info control image should be added behind the checkbox for this layer. This info control allows user to toggle information for this layer, when using Kaboum info tool. |
Example |
|
type:
Type |
String. fixed values. see example. Case sensitive |
Description |
The type of control for the layer. Either a checkbox or a select entry |
Example |
'CHECKBOX' or 'SELECT' |
css_style:
Type |
String. Use an empty string when no CSS style is required. |
Description |
The name of a CSS class style to be used when rendering the component. The CSS class will be put into a <SPAN> tag |
Example |
'MyClass' |
|
example 1 |
<script>kNewTheme("departements","Départements Français",true,true,"CHECKBOX",'tiny');</script>
|
example 2 |
<script>kNewTheme("ortho","Orthophoto",false,false,"SELECT",'');kLastSelectTheme();</script> |
|
4.3 The legend control
4.3.1 Introduction
What's a map without a legend ? Kaboum Javascript API provides users with a way to automatically opens a legend window directly plugged to Mapserver. It is so compulsory to define such a LEGEND ojbect into the Mapfile displayed by Kaboum.
4.3.2 How to create a legend control: the kgetLegend() method
You just have to decide what kind of component you want to open the legend window (link, button, form element, etc.) and call the kGetLegend() when user interacts with it.
Example 1: <a href="#" onclick="kGetLegend();">Display the map legend</a>
Example 2: <input type="button" name="legendBut" value="display the legend" onclick="kGetLegend();"/>
4.4 The scale control
4.4.1 Introduction
What's a map without a scale ?... well, Mapserver does provide a scale object displaying a nice, customizable scale. But Kaboum Javascript API provides a component to display a dynamic, numeric scale. User can change the scale value and the map is automatically zoomed to this new scale.
4.4.2 How to integrate a scale control: the kNewScale(...) method
The scale control is a text field that will contain the current map scale each time a new map is displayed by kaboum. To create this text field, use the method below:
kNewScale(scaleText, style):
Description |
Creates a textfield scale control, with the given text in front of it. This textfield is dynamic. If user modifies its value and validates (enter), kaboum refreshes the map to zoom to the new scale. |
Parameters |
scaleText:
Type |
String |
Description |
The text that will be apended in front of the textfield |
Example |
'1:' |
style:
Type |
String. |
Description |
The name of a CSS class style to be used when rendering the component. |
Example |
'invisible_borders' |
|
example |
<script>kNewScale("1:","");</script>
|
|
4.5. The coordinates control
4.5.1 Introduction
Kaboum applet tracks down mouse movements on the map and converts them into map coordinates by applying the exact same algorithm as the mapserver one to transform between screen coordinates (x-y integers) into map, unit-dependent coordinates.
It is possible to integrate these coordinates into the HTML page thanks to the coordinates control. (Note: coordinates are also displayed in the browser's status bar). Developer can then use these coordinates for any geographic-related task.
Coordinates are displayed into textfield Javascript components.
4.5.2 How to integrate coordinates control: the kNewXCoordinate(...) and kNewYCoordinate(...) methods
These 2 methods are strictly the same. One handles the X-coordinate coming from Kaboum, the other handles the y-coordinate.
kNewXCoordinate(coordText, style, size):
Description |
Creates a textfield control that will display the x-coordinate of the mouse moving on a Kaboum map. To enable this mechanism, sets the kaboum init parameter KABOUM_SEND_POSITION_COORDINATES_TO_JS to "true" |
Parameters |
scaleText:
Type |
String |
Description |
The text that will be apended in front of the textfield |
Example |
'x coord:' |
style:
Type |
String. |
Description |
The name of a CSS class style to be used when rendering the component. |
Example |
'invisible_borders' |
size:
Type |
integer |
Description |
The size of the textfield |
Example |
8 |
|
example |
<script>kNewXCoordinate("x:","", 8 );</script>
|
|
kNewYCoordinate(coordText, style, size):
Description |
Creates a textfield control that will display the y-coordinate of the mouse moving on a Kaboum map. To enable this mechanism, sets the kaboum init parameter KABOUM_SEND_POSITION_COORDINATES_TO_JS to "true"
|
Parameters |
scaleText:
Type |
String |
Description |
The text that will be apended in front of the textfield |
Example |
'y coord:' |
style:
Type |
String. |
Description |
The name of a CSS class style to be used when rendering the component. |
Example |
'invisible_borders' |
size:
Type |
integer |
Description |
The size of the textfield |
Example |
8 |
|
example |
<script>kNewXCoordinate("y:","", 8 );</script>
|
|

5. kaboum.js Reference
5.1. Structure
This file is organized as follow:
- "Public" and "private" global variables declaration and initalisation: public variables can be overloaded by developers. private one should not, though no control is performed to ensure private variables are not overloaded.
- Browser sniffing code
- kaboumCommand() and kaboumResult() methods implementation
- Public methods to create themes, buttons, scale, legend, etc. objects
5.2. Public Global variables
These variables can be overloaded by developers to customize their kaboum environment. To do so, creates a new script and overload variables in it. Do not change or edit kaboum.js directly !
kButtonType:
Description |
the file extension for toolbar images buttons (kNewButton object). All buttons must have the same extension.
the image type must be supported by browsers. (use gif, png or jpg image types). |
Type |
String |
Public |
this parameter can be overloaded by developer to change buttons type |
Default value |
.gif |
|
kThemeAutoReload:
Description |
Boolean telling if the map should be reloaded each time a theme is selected/deselected. If false, developer must provide a button allowing user to reload kaboum map. |
Type |
boolean |
Public |
this parameter can be overloaded by developer to change default behaviour |
Default value |
true |
|
kinfoImgSrc:
Description |
the path, relative or absolute, to an image used to control information visibility of a theme. This image should be small enough to integrate easily in front of a text.
Javascript code generates a second image name based on this parameter's value. The first image is used when information is not available for the layer, the second image is used when information is available. The second image must exist in the same folder as the first one, and its name must follow this format:
<first_image_name>_clicked.gif ("_clicked" must be present in the image's name before the file extension.) |
Type |
String |
Public |
Developer can overload this parameter to customize theme's info images. |
Default value |
"images/infolayer.gif" (this image: ) |
|
kCurrentExtent:
Description |
the current kaboum map extent. Used for debug and for developper needing it |
Type |
String |
Public |
Its value should not be changed, but used by developer who wants to control map extent for some reason |
Default value |
empty string |
|
kActiveThemes:
Description |
The list of comma-separated active themes names. Used for debug mainly, and to control what are the active themes |
Type |
String of themes' names. |
Public |
Its value should not be changed, but used by developer who wants to control map extent for some reason |
Default value |
empty string |
|
kServletPath:
Description |
The URL of the application server (servlet or something else) processing server actions, like vector objects management |
Type |
URL |
Public |
Its value should be defined in user-specific javascript when server processing is needed |
Default value |
false (Javascript dynamic typing allows this value to be changed into a String by user). |
|
kEditionMode:
Description |
Tells if kaboum will deal with vector, editable objects. If true, the kaboum_edition.js file must be linked in the page containing kaboum |
Type |
boolean |
Public |
Its value should be true if vector objects are used. (overload this parameter in user-specific JS). |
Default value |
false |
|
kPrintURL:
Description |
The url of the print layout page, allowing user to choose print settings for the kaboum map |
Type |
URL to a valid HTML page |
Public |
Developer can provide its own printing page (specific layout for instance), provided the print mechanism is conform to the original one. The simplest way to customize the print settings page is to edit the original one and to modify its layout |
Default value |
print.html |
|
kPrintDirect:
Description |
Tells if map print should be made directly, without poping print.html page. |
Type |
boolean |
Public |
If true, developer should provide print properties himself (title, comment, orientation, paper size, etc) |
Default value |
false |
|
kHelpURL:
Description |
The url of the help page. this page is opened when the help button is clicked. Any HTML page is ok. |
Type |
URL to a valid HTML page |
Public |
Developer can provide its own Help page |
Default value |
help.html |
|
kQueryURL:
Description |
The url of the attributes query page. this page is opened when the query button is clicked. Any HTML page is ok. |
Type |
URL to a valid HTML page |
Public |
Developer can provide its own query page |
Default value |
query.html |
|
kPrintComment:
Description |
The text used as a comment when printing kaboum map.
This text can be entered by the user if the print.html page is used to control print settings |
Type |
String |
Public |
If the default print.html page is used, this parameter is entered by the user |
Default value |
empty string |
|
kPrintTitle:
Description |
The text used as a title when printing kaboum map.
This text can be entered by the user if the print.html page is used to control print settings |
Type |
String |
Public |
If the default print.html page is used, this parameter is entered by the user |
Default value |
empty string |
|
kPrintPortrait:
Description |
Tells if user has choosen the portrait mode when printing kaboum map (see print function)
This value can be choosen by the user if the print.html page is used to control print settings |
Type |
boolean |
Public |
|
Default value |
false |
|
kPrintLegend:
Description |
Tells if user has choosen to include legend when printing kaboum map (see print function)
This value can be choosen by the user if the print.html page is used to control print settings |
Type |
boolean |
Public |
|
Default value |
false |
|
|
5.3. Private Global variables
These variables should not be changed by developpers. Their value could be retrieved or used in specific code.
kButtonArray:
Description |
Global array containing all buttons (kNewButton objects) composing the toolbar. these buttons can be placed anywhere in the applet's page |
Type |
Array of kNewButton objects |
Private |
|
|
kThemeArray:
Description |
Global array containing all themes (kNewTheme objects) created in the page. |
Type |
Array of kNewTheme objects |
Private |
|
|
kInfoArray:
Description |
Global array containing all information buttons (kInfoTheme objects) created in the page. For each theme, an info button can created |
Type |
Array of kInfoTheme objects |
Private |
|
|
kButtonImages:
Description |
Global array containing all images composing buttons. Each toolbar button can be configured with an image |
Type |
Array of images objects |
Private |
|
|
kThemeSelectWritten:
Description |
Boolean telling if the Javascript Select object containing types whose type is select is written or not. Reset each time lastSelectTheme() is called, to allow a new select object containing other themes to be created |
Type |
boolean |
Private |
|
Default value |
false |
|
kFirstMap:
Description |
Boolean telling that the first kaboum map is to be retrieved. If so, stores the original mapextent for later use. |
Type |
Boolean |
Private |
|
Default value |
true |
|
kInitialExtent:
Description |
the initial kaboum map extent. Stored when the first map is retrieved, to use in navigation and "home" command. |
Type |
String |
Private |
|
Default value |
empty string |
|
kGASIncluded:
Description |
the boolean telling if the page is used by the GAS. If so, a third js page, kaboum_gas.js, must be included in the map view and sets this value to true.
Used only in the GAS application |
Type |
boolean |
Private |
|
Default value |
false |
|
kScaleField:
Description |
the textfield object used to display the map scale, if it was defined by a call to kNewScale() |
Type |
String |
Private |
|
Default value |
empty string |
|
kxCoordField:
Description |
the textfield object used to display the x current position of the mouse in the map, if it was defined by a call to kNewXCoordinate() |
Type |
String |
Private |
|
Default value |
empty string |
|
kyCoordField:
Description |
the textfield object used to display the y current position of the mouse in the map, if it was defined by a call to kNewYCoordinate() |
Type |
String |
Private |
|
Default value |
empty string |
|
kPrintComment:
Description |
The text choosen by the user as a comment when printing kaboum map |
Type |
String |
Private |
|
Default value |
empty string |
|
kPrintComment:
Description |
The text choosen by the user as a comment when printing kaboum map |
Type |
String |
Private |
|
Default value |
empty string |
|
kPrintTitle:
Description |
The text choosen by the user as a title when printing kaboum map |
Type |
String |
Private |
|
Default value |
empty string |
|
kPrintPortrait:
Description |
Tells if user has choosen the portrait mode when printing kaboum map (see print function) |
Type |
boolean |
Private |
|
Default value |
false |
|
kPrintLegend:
Description |
Tells if user has choosen to include legend when printing kaboum map (see print function) |
Type |
boolean |
Private |
|
Default value |
false |
|
5.4 Public methods
5.4.1 kItemQueryNoResult() method
Beside methods describe on chapter 4, allowing to create predefined controls to drive Kaboum applet, there are some public methods available to developers to realize custom tasks:
kItemQueryNoResult():
Description |
When Kaboum applet is in the mode ITEM_NQUERYMAP, if no result is returned by the query, the applets returns a command to the client. This command is catched by the kItemQueryNoResult() method. Developers can overload this method to provide custom message to user or to implement a special mechanism.
The default mechanism of the kItemNoQueryResult() method is to popup a Javascript message box containing the following message:
"Mapserver did not return any result for the query."
The developer can overload this method, for instance to change the default message language.
|
Parameters |
This method has no parameters |
example |
//in developer's Javascript code:
kItemQueryNoResult() {
alert("la requete n'a renvoyé aucun resultat");
} |
|
5.4.2 Initialisation method: kInit()
When the kaboum.js code is executed, the kInit() method is called when the page is loaded (onLoad() event loader). Some commands are then sent to kaboum to initialize the list of active layers and their info status.
If the developer implements its own onLoad event handler, he must call kInit() in this method in order for the applet to work correctly.
